この記事で分かること
- Spring RestTemplateの使い方 ベストプラクティス(GET / POSTメソッド)
- それぞれのケースにおいて、コピペで使えるテンプレート
- Queryパラメータ / Path変数 / Content-Type / Autorizationヘッダ の有無による呼び出し方について
この記事では、Spring bootでRestTemplateを使ってAPIを呼び出す際のベストプラクティスを紹介します。
一口にAPIを呼び出すと言っても、HTTPメソッドの違いや、パラメータの有無、パラメータ形式の違い(JSON/XML)、ヘッダの有無などにより微妙に呼び出し方が違っており、毎回使い方を検索しているという人が多いと思います。
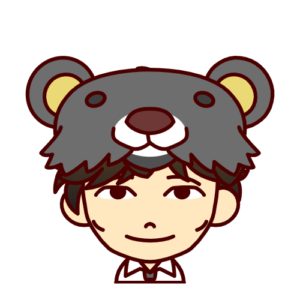
いつも使い方を忘れるので、いい加減自分でまとめた方が良いんじゃないかと思い記事を書きました(笑
今回は、よく使われるGET / POSTメソッドについて、コピペで使えるようなテンプレート一覧を用意しました。
Spring boot + RestTemplate でAPIを呼び出す際は、このページの内容をぜひ参考にしていただければと思います。
目次
RestTemplateの呼び出しテンプレート
RestTemplateのドキュメントは以下を参照
https://spring.pleiades.io/spring-framework/docs/current/javadoc-api/org/springframework/web/client/RestTemplate.html
GET (パラメータなし)
public void get() {
RequestEntity<Void> request = RequestEntity
.get("http://localhost:8080/api/get")
.build();
ResponseEntity<String> response = restTemplate.exchange(request, String.class);
}
GET (パラメータあり)
public void getWithParams() {
URI uri = UriComponentsBuilder.fromUriString("http://localhost:8080/api/get")
.queryParam("param1", "value1")
.build().toUri();
RequestEntity<Void> request = RequestEntity
.get(uri)
.build();
ResponseEntity<String> response = restTemplate.exchange(request, String.class);
}
POST (パラメータなし)
public void post() {
RequestEntity<Void> request = RequestEntity
.post("http://localhost:8080/api/post")
.build();
ResponseEntity<String> response = restTemplate.exchange(request, String.class);
}
POST (PathVaribaleあり)
public void postWithPathVariable() {
URI uri = UriComponentsBuilder.fromHttpUrl("http://localhost:8080/api/{pathVariable}/post")
.buildAndExpand(Collections.singletonMap("pathVariable", "sample"))
.toUri();
RequestEntity<Void> request = RequestEntity
.post(uri)
.build();
ResponseEntity<String> response = restTemplate.exchange(request, String.class);
}
POST (Content-Type: application/x-www-form-urlencoded)
public void postWithUrlParams() {
MultiValueMap<String, String> map = new LinkedMultiValueMap();
map.add("param1", "value1");
map.add("param2", "value2");
RequestEntity<MultiValueMap<String, String>> request = RequestEntity
.post("http://localhost:8080/api/post-url-encoded")
.contentType(MediaType.APPLICATION_FORM_URLENCODED)
.body(map);
ResponseEntity<String> response = restTemplate.exchange(request, String.class);
}
POST (Content-Type: application/json)
public void postWithJson(ApiRequest apiRequest) {
RequestEntity<ApiRequest> request = RequestEntity
.post("http://localhost:8080/api/post-json")
.contentType(MediaType.APPLICATION_JSON)
.body(apiRequest);
ResponseEntity<ApiResponse> response = restTemplate.exchange(request, ApiResponse.class);
}
POST (Authorizationヘッダあり)
public void postWithHeader() {
RequestEntity<Void> request = RequestEntity
.post("http://localhost:8080/api/post-header")
.header(HttpHeaders.AUTHORIZATION, "Bearer token")
.build();
ResponseEntity<String> response = restTemplate.exchange(request, String.class);
}
POST (全部入り)
public void postWithAll(ApiRequest apiRequest) {
URI uri = UriComponentsBuilder.fromHttpUrl("http://localhost:8080/api/{pathVariable}/post")
.buildAndExpand(Collections.singletonMap("pathVariable", "sample"))
.toUri();
RequestEntity<ApiRequest> request = RequestEntity
.post(uri)
.contentType(MediaType.APPLICATION_JSON)
.header(HttpHeaders.AUTHORIZATION, "Bearer token")
.body(apiRequest);
ResponseEntity<ApiResponse> response = restTemplate.exchange(request, ApiResponse.class);
}
まとめ
要点
- RequestEntity と exchangeメソッドを使ってAPIを呼び出す方法がオススメ
- UriComponentsBuilder を使ってURIを作成すると可読性が良くなる
- JSONで送受信するREST APIは、Jacksonのサポートにより自動的にDTOを生成できるため便利
RestTemplateには、getForObjectやpostForEntityといったメソッドも用意されていますが、exchangeメソッドを使った方法の方が色々な場面に対応しやすいのでオススメしたいです。
現時点でベストと思われる方法を紹介しましたが、
もし、もっと良い方法が見つかりましたら、記事を随時アップデートしていくつもりです。
最後まで読んで頂きありがとうございました。
もしこの記事が参考になりましたら、以下のボタンからフィードバックを頂けるとありがたいです!